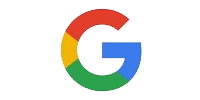
0
+
Google Reviews

0
+
MEAN stack refers to a collection of JavaScript based technologies used to develop web applications. MEAN is an acronym for MongoDB, ExpressJS, AngularJS and Node. js. From client to server to database, MEAN is full stack JavaScript. This course introduces development techniques that capitalise on the strengths of every layer in the MEAN stack, using a simple shopping list application project that has a Backend server side Api built with Node , Express and MongoDB and a Frontend client built with angular 6 that will exchange with the backend Api . Data will be exchanged between a browser based client and an API backend service . You will learn the essential concepts of the MEAN stack
Duration of Training : 40 hrs
Batch type : weekdays /weekends/ Customized Batches
Mode of Training: Offline / Online / Corporate Training
Projects Given : 2 Projects minimum
Trainer Profile : Experienced Faculty from IT Industry
Projects | Assignment | Scenarios and Used Case Studies
Node.js Background
Getting Node.js
Demo: Installing Node on Linux/Windows with NVM
Node’s Event Loop
Node Conventions for Writing Asychronous Code
Modules, require() and NPM
Introduction, Accessing Built-in Modules
Finding 3rd Party Modules via NPM
Introduction
Setting up Express
Running Express
Debugging Options
Static Files – Public Directory
Introduction, Making Web Requests in Node
Building a Web Server in Node
Demo: Building a Web Server in Node
Introduction
Navigation
Routing
Rendering
Separate Files
Router Functions
Introduction to Authentication
Auth Routes
Passport
Local Strategy
Introduction
Events and the EventEmitter class
Readable and Writable Streams, the Pipe function
Demo: Readable and Writable Streams
Demo: Piping Between Streams
The Process Object
Demo: The Process object
Interacting with the File System
Demo: Interacting with the File System
What is a Buffer?
The OS Module
Realtime Interaction with Socket.IO
Demo: Socket.IO
Testing and Debugging
Introduction, The Assert Module
Demo: The Assert Module
Testing with Mocha and Should.js
Demo: Mocha and Should.js
Scaling Your Node Application
Introduction, The Child Process Module
Demo: The “exec” function
Demo: The “spawn” function
Demo: The “fork” function
Scaling with Node’s Cluster Module
Demo: Building a Clustered Web Server
Introduction
Downloading
Package Content
First Run
Command Line Options
Verify Server
Getting Help in the Shell
Saving Data
Introduction
Overview
Storage
BSON
Saving Documents
Collections
Document Id
ObjectId
Insert
Insert with Id
Complex Document
Save Danger
Update Command
Update Demo
Set Operator
Unset Operator
Rename Operator
Push Operator
Pull Operator
Pop Operator
Array Type
Multi Update
Find And Modify
Query With Sort
Finding Documents
Introduction
Overview
find()
Equality
Projection
Comparison
$not
$in
Arrays
$all
$nin
Dot Notation
Sub-Document
null and $exists
And
Cursor
sort()
limit()
skip()
findOne()
Deleting Document
remove
deleteOne
deleteMany
Indexing
Introduction
Overview
Scan Is Bad
Index Theory
Sort Uses Index
Index Types
Create Index
system.indexes collection
explain()
dropIndex()
Index Name
Getting Started with Angular
Introduction
Introduction to TypeScript
Comparing Angular to AngularJS
A Conceptual Overview of Angular
Getting Started with the Angular CLI
Bootstrapping an Angular App
A Brief Look at the App Module
Accessing Static Files
Creating and Communicating Between Angular Components
Introduction
Creating Your First Data-bound Component
Using External Templates
Communicating with Child Components Using @Input
Communicating with Parent Components Using @Output
Using Template Variables to Interact with Child Components
Styling Components
Exploring Angular’s CSS Encapsulation
Adding a Site Header
Exploring the Angular Template Syntax
Introduction
Interpolation, Property Bindings, and Expressions
Event Bindings and Statements
Repeating Data with ngFor
Handling Null Values with the Safe-Navigation Operator
Hiding and Showing Content with ngIf
Hiding Content with the [Hidden] Binding
Hiding and Showing Content with ngSwitch
Styling Components with ngClass
Styling Components with ngStyle
Creating Reusable Angular Services
Introduction
Why We Need Services and Dependency Injection
Creating Your First Service
Wrapping Third Party Services
Routing and Navigating Pages
Introduction
Adding Multiple Pages to Your App
Adding Your First Route
Accessing Route Parameters
Linking to Routes
Navigating from Code
Guarding Against Route Activation
Guarding Against Route De-activation
Pre-loading Data for Components
Styling Active Links
Collecting Data with Angular Forms and Validation
Introduction
Using Models for Type Safety
Creating Your First Template-based Form
Using the Data from Your Template-based Form
Validating Template-based Forms
Creating Your First Reactive Form
Validating Reactive Forms
Using Multiple Validators in Reactive Forms
Diving Deeper into Template-based Forms
Editing Data with Two-way Bindings
Diving Deeper into Reactive Forms
Creating Custom Validators
Communicating Between Components
Introduction
Passing Data into a Child Component
Passing Data out of a Child Component
Reusing Components with Content Projection
Introduction
Content Projection
Multiple Slot Content Projection
Displaying Data with Pipes
Introduction
Using Built-in Pipes
Creating a Custom Pipe
Sorting and Filtering Overview
Creating a Filtering Display
Filtering Data
Sorting Data
Summary
Understanding Angular’s Dependency Injection
Introduction
Using Third Party Global Services
Angular Dependency Injection Lookup
Using Angular’s InjectionToken
Using Angular’s @Inject Decorator
The useClass Provider
The useExisting and useFactory Providers
Creating Directives and Advanced Components in Angular
Introduction
Implementing the Session Search
Adding jQuery
Creating a Modal Component
Fixing Template Parse Errors
Creating Directives
Binding an ID
Routing to the Same Component
Using the @ViewChild Decorator
Creating Settings on Components
Communicating with the Server Using HTTP, Observables
Introduction
Preparing to Store Data on the Server
Moving Data Storage to the Server
Listening to Resolved Data Changes
Using POST and PUT
Using QueryString Parameters
Using DELETE
Integrating Authentication with the Server
Persisting Authentication Status Across Page Refreshes
Saving User Data to the Server
Implementing Logout
Unit Testing Your Angular Code
Introduction
Installing Karma6m 16s
Unit Testing Services8m 16s
Testing Mock Calls5m 44s
Testing Components with Isolated Tests
Testing Angular Components with Integrated Tests
Introduction
Setting up for Integrated Tests
Testing Components with Deep Integrated Tests
Creating Mock Services
Using DebugElement
Testing Components with Shallow Integrated Tests
Taking an Angular App to Production
Introduction
Linting Overview
Installing TSLint in VSCode
Using TSLint with VSCode
Linting from the Command Line
Going to Production – Overview
Creating your First Build
Basic Deployment
Build Flags
The Effects of Prod Mode
Optimistic Bundle Downloading
+91 8882400500
I had undergone oracle DBA course under Chetan sir's Guidance an it was a very good learning experience overall since they not only provide us with theoretical knowledge but also conduct lot of practical sessions which are really fruitful and also the way of teaching is very fine clear and crisp which is easier to understand, overall I had a great time for around 2 months, they really train you well.also make it a point to clear all your doubts and provide you with clear and in-depth concepts hence hope to join sometime again
I have completed Oracle DBA 11g from Radical technology pune. Excellent trainer (chetna gupta). The trainer kept the energy level up and kept us interested throughout. Very practical, hands on experience. Gave us real-time examples, excellent tips and hints. It was a great experience with Radical technologies.
Linux learning with Anand sir is truly different experience... I don't have any idea about Linux and system but Anand sir taught with scratch...He has a great knowledge and the best trainer...he can solve all your queries related to Linux in very simple way and giving nice examples... 100 to Anand Sir.
I had a wonderful experience in Radical technologies where i did training in Hadoop development under the guidance of Shanit Sir. He started from the very basic and covered and shared everything he knew in this field. He was brilliant and had a lot of experience in this field. We did hands on for every topic we covered, and that's the most important thing because honestly theoretical knowledge cannot land you a job.
I have recently completed Linux course under Anand Sir and can assuredly say that it is definitely the best Linux course in Pune. Since most of the Linux courses from other sources are strictly focused on clearing the certification, they will not provide an insight into real-world server administration, but that is not the case with Anand Sir's course. Anand Sir being an experienced IT infrastructure professional has an excellent understanding of how a data center works and all these information is seamlessly integrated into his classes.
1. Basic user account management (creating, modifying, and deleting users).
2. Password resets and account unlocks.
3. Basic file system navigation and management (creating, deleting, and modifying files and directories).
4. Basic troubleshooting of network connectivity issues.
5. Basic software installation and package management (installing and updating software packages).
6. Viewing system logs and checking for errors or warnings.
7. Running basic system health checks (CPU, memory, disk space).
8. Restarting services or daemons.
9. Monitoring system performance using basic tools (top, df, free).
10. Running basic commands to gather system information (uname, hostname, ifconfig).
1. Intermediate user account management (setting permissions, managing groups).
2. Configuring network interfaces and troubleshooting network connectivity issues.
3. Managing file system permissions and access control lists (ACLs).
4. Performing backups and restores of files and directories.
5. Installing and configuring system monitoring tools (Nagios, Zabbix).
6. Analyzing system logs for troubleshooting purposes.
7. Configuring and managing software repositories.
8. Configuring and managing system services (systemd, init.d).
9. Performing system updates and patch management.
10. Monitoring and managing system resources (CPU, memory, disk I/O).
1. Advanced user account management (LDAP integration, single sign-on).
2. Configuring and managing network services (DNS, DHCP, LDAP).
3. Configuring and managing storage solutions (RAID, LVM, NFS).
4. Implementing and managing security policies (firewall rules, SELinux).
5. Implementing and managing system backups and disaster recovery plans.
6. Configuring and managing virtualization platforms (KVM, VMware).
7. Performance tuning and optimization of system resources.
8. Implementing and managing high availability solutions (clustering, load balancing).
9. Automating system administration tasks using scripting (Bash, Python).
10. Managing system configurations using configuration management tools (Ansible, Puppet).
1. Learning basic shell scripting for automation tasks. 2. Understanding file system permissions and ownership. 3. Learning basic networking concepts (IP addressing, routing). 4. Learning how to use package management tools effectively. 5. Familiarizing with common Linux commands and utilities. 6. Understanding basic system architecture and components. 7. Learning basic troubleshooting techniques and methodologies. 8. Familiarizing with basic security principles and best practices. 9. Learning how to interpret system logs and diagnostic output. 10. Understanding the role and importance of system backups and restores.
1. Advanced scripting and automation techniques (error handling, loops).
2. Understanding advanced networking concepts (VLANs, subnetting).
3. Familiarizing with advanced storage technologies (SAN, NAS).
4. Learning advanced security concepts and techniques (encryption, PKI).
5. Understanding advanced system performance tuning techniques.
6. Learning advanced troubleshooting methodologies (root cause analysis).
7. Implementing and managing virtualization and cloud technologies.
8. Configuring and managing advanced network services (VPN, IDS/IPS).
9. Implementing and managing containerization technologies (Docker, Kubernetes).
10. Understanding enterprise-level IT governance and compliance requirements.
1. Designing and implementing complex IT infrastructure solutions. 2. Architecting and implementing highly available and scalable systems. 3. Developing and implementing disaster recovery and business continuity plans. 4. Conducting security audits and vulnerability assessments. 5. Implementing and managing advanced monitoring and alerting systems. 6. Developing custom automation solutions tailored to specific business needs. 7. Providing leadership and mentorship to junior team members. 8. Collaborating with other IT teams on cross-functional projects. 9. Evaluating new technologies and making recommendations for adoption. 10. Participating in industry conferences, workshops, and training programs.
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
Abu Dhabi
(Our Team will call you to discuss the Fees)
(Our Team will call you to discuss the Fees)